Installing Lambda
Perform the following procedure to enable Lambda monitoring from AWS.
- Ensure Cloud Watch logs are enabled for your lambda function, In addition AWS creates a Log Group for every lambda function. Ensure that this log group has the same name as your lambda function. If they are not the same, you will not see some data on the SixthSense Lambda monitoring page.
- To capture the Lambda source invocation on your SixthSense Lambda monitoring page, you must add specific lines of code according to your runtime.
Python
Node JS
Ruby
Java
Add a few dependencies as follows to your class path.
- aws-lambda-java-core
- aws-lambda-java-events
- gson
- Maven pom.xml example
- Create a new Class
- Tweak your lambda handler class to invoke the SSLambda class log function.
Example:
Installing Lambda
Ensure that you have configured AWS. For more information, see Installing AWS.
Once you have configured AWS, you must install Lambda.
- Login to the SixthSense portal.
- Click Integrations on the left pane.
- Click Configure in the Amazon Web Services card available.
If you have already configured services the AWS Configurations page similar to the following appears. If you have not configured services, see Adding a configuration
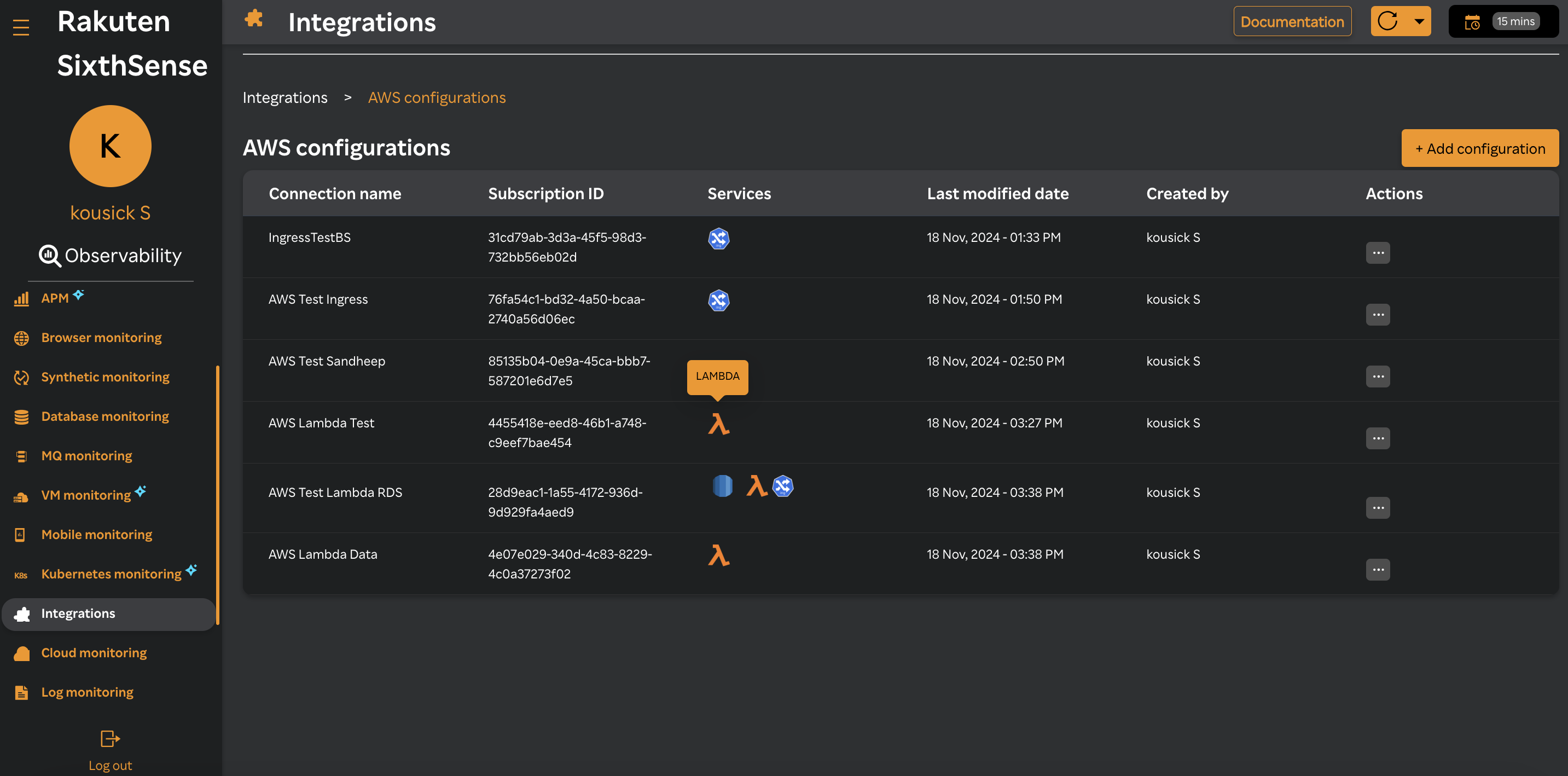
- Click the ellipse icon against a connection name.
- Select View services from the list.
A screen similar to the following appears with no services installed.
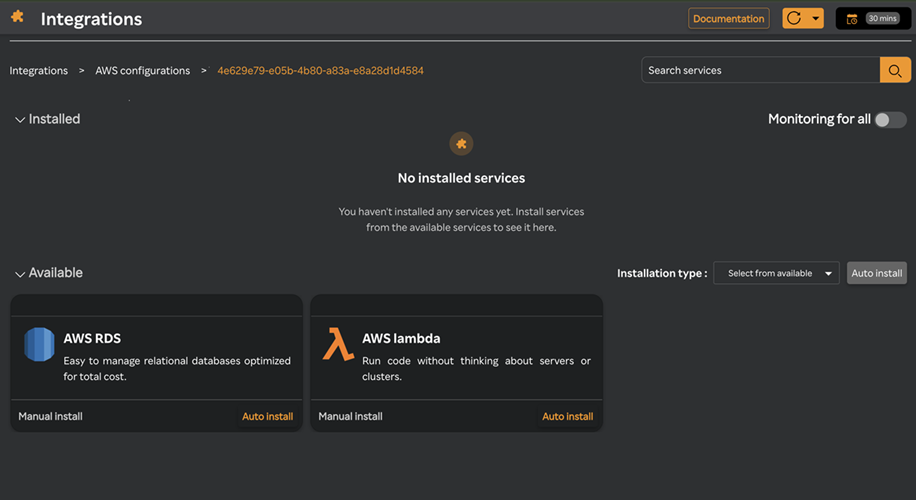
- Click Manual Install in the AWS Lambda card to install it manually or click Auto Install to automatically install the Lambda service.
note
Auto install monitors all functions.
- If you choose Manual Install, the following screen appears.
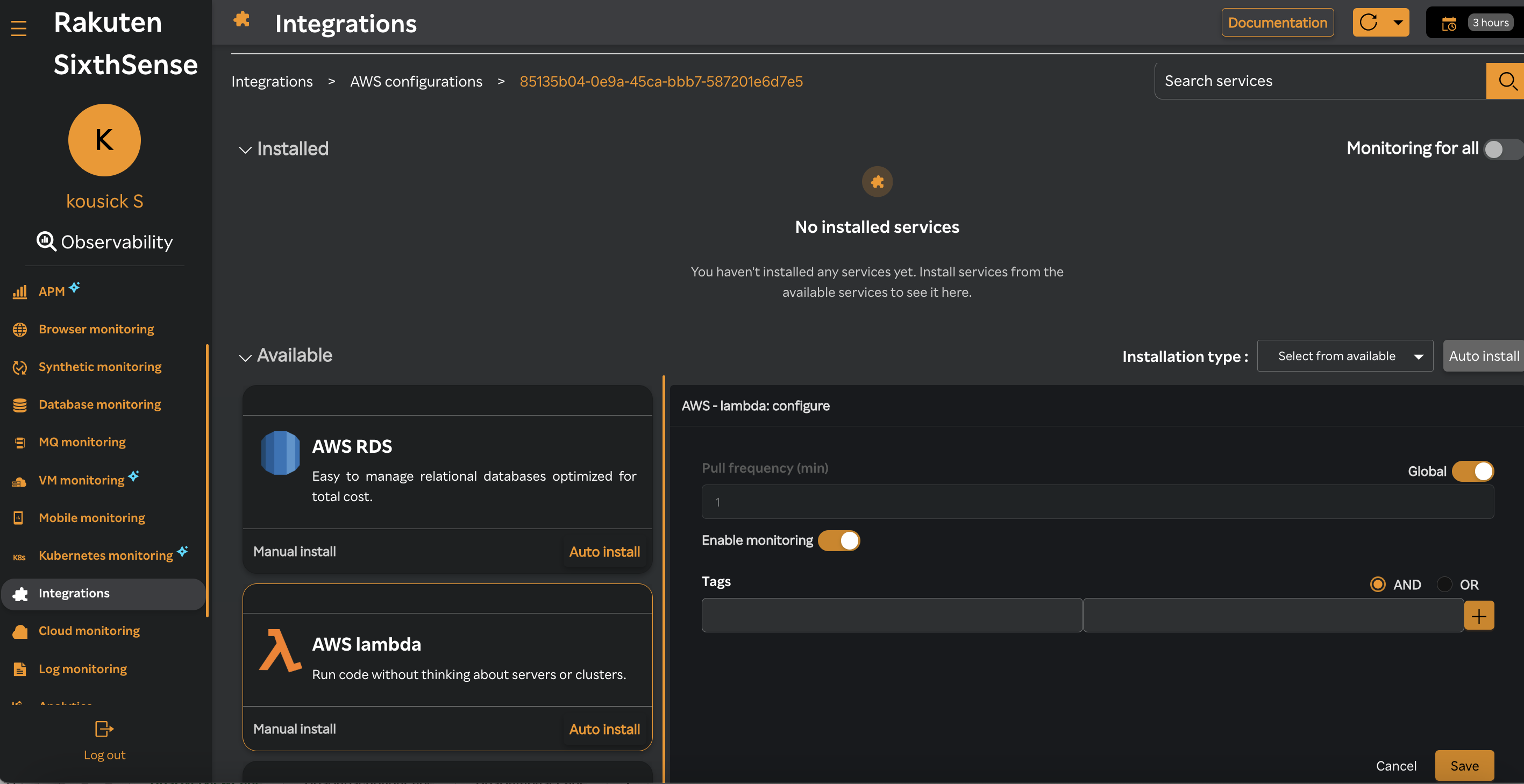
- Fill in the following fields.
Field | Description |
---|---|
Pull Frequency(min) | This frequency can be set to global for all AWS services at the tenant level. You can define AWS service specific pulling frequency. Global pulling frequency can be 5 mins. Note: If AWS service specific pulling frequency is configured, this will have higher precedence over Global pulling frequency. |
Global toggle button | Disabling Global will take the pull frequency set at the service level. |
Enable Monitoring | Allows you to monitor all the services. |
Tags | Click + to add multiple tags against each row. Rows are added to add more tags. For more information, see Tagging functions. |
- Click Save.
You will see that Lambda is configured as in the following screen.
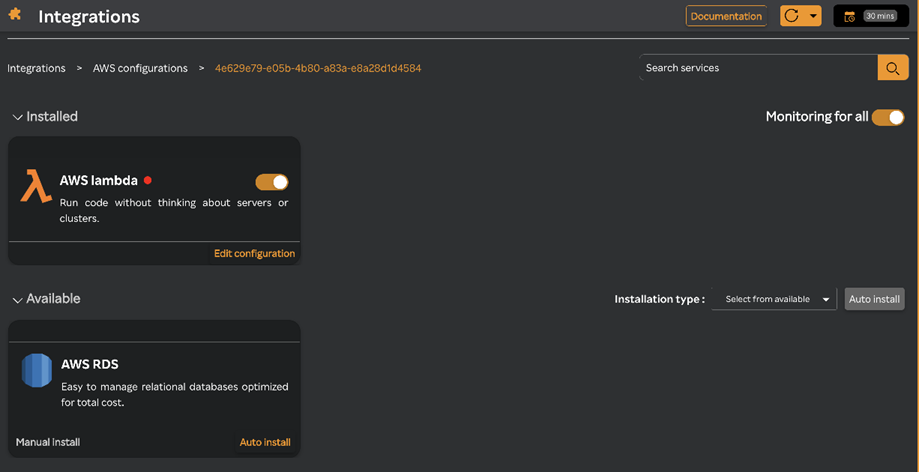
The connection is verified with the AWS credentials and you will see the AWS Lambda card under the Installed header.
Adding a configuration
- Navigate to the AWS configurations page.
- Click + Add configuration if you haven't added the AWS configuration.
Fill in the following fields.
Field name | Description |
---|---|
Connection name | The identifier or label assigned to a specific connection when connecting to a function. |
Access key ID | A unique identifier that is public and can be used to identify the IAM user. |
Secret access key | A secret string that is used together with the Access Key ID to sign programmatic requests. |
Pull frequency (min) | Pull frequency is how often a system, application, or service requests or "pulls" data from another system or source. Can be at subscription or service level. |
- Click Configure.
Tagging functions
Use tags for monitoring a particular or multiple functions. You can also have mutlitple tags for a function using the AND or OR conditions. Note that you can use only one of the conditions while adding multiple tags.
While adding tags enter the Key value for the function. The value could be the capability name followed by a string.
You must also enter the value that is required for picking the specified values from the function. You can use the logical AND/OR operators for the values you select.
note
Tags are available only for manual installation.
Editing a Lambda configuration.
- Login to the SixthSense portal.
- Click Integrations on the left pane.
- Click Configure in the Amazon Web Services card available.
The AWS Configurations page similar to the following appears.
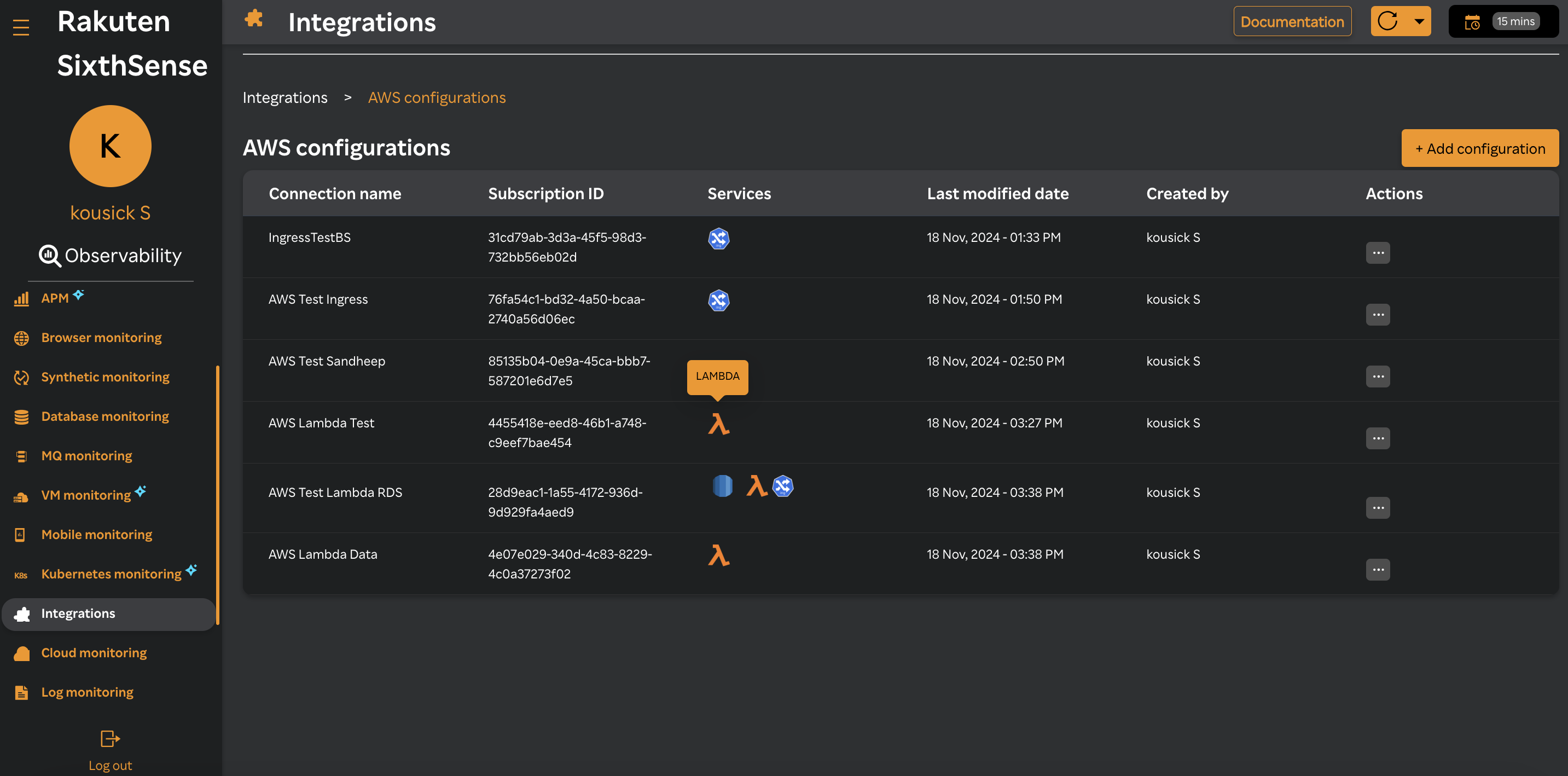
- Click the
icon against a connection name.
- Select View services from the list.
- Select Edit configuration.
- Edit the required fields.
- Click Configure.